Windows Registry Editor Version 5.00
[HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Internet Explorer\Main\FeatureControl\FEATURE_BROWSER_EMULATION]
"Fitbit Connect.exe"=dword:000022b8
For those familiar with the Windows registry the above should be enough. For those not familiar, copy and paste the above into notepad, save as a file named "fitbit.reg", and then double click the reg file and say 'Yes' to the prompt. Hopefully in the final release of Windows 8.1 this won't be an issue.
Thursday, August 29, 2013
FitBit and WebOC Application Compatibility Errors
I just got a FitBit One from my wife. Unfortunately I had issues running their app on my Windows 8.1 Preview machine. But I recognized the errors as IE compatibility issues, for instance an IE dialog popup from the FitBit app telling me about an error in the app's JavaScript. Given my previous post on WebOC versioning you may guess what I tried next. I went into the registry and tried out different browser mode and document mode versions until I got the FitBit software running without error. Ultimately I found the following registry value to work well ('FitBit connect.exe' set to DWORD decimal 8888).
Thursday, August 15, 2013
Moving PowerShell data into Excel
PowerShell nicely includes ConvertTo-CSV and ConvertFrom-CSV which allow you to serialize and deserialize your PowerShell objects to and from CSV. Unfortunately the CSV produced by ConvertTo-CSV is not easily opened by Excel which expects by default different sets of delimiters and such. Looking online you'll find folks who recommend using automation via COM to create a new Excel instance and copy over the data in that fashion. This turns out to be very slow and impractical if you have large sets of data. However you can use automation to open CSV files with not the default set of delimiters. So the following isn't the best but it gets Excel to open a CSV file produced via ConvertTo-CSV and is faster than the other options:
Param([Parameter(Mandatory=$true)][string]$Path);
$excel = New-Object -ComObject Excel.Application
$xlWindows=2
$xlDelimited=1 # 1 = delimited, 2 = fixed width
$xlTextQualifierDoubleQuote=1 # 1= doublt quote, -4142 = no delim, 2 = single quote
$consequitiveDelim = $False;
$tabDelim = $False;
$semicolonDelim = $False;
$commaDelim = $True;
$StartRow=1
$Semicolon=$True
$excel.visible=$true
$excel.workbooks.OpenText($Path,$xlWindows,$StartRow,$xlDelimited,$xlTextQualifierDoubleQuote,$consequitiveDelim,$tabDelim,$semicolonDelim, $commaDelim);
See Workbooks.OpenText documentation for more information.
Saturday, August 10, 2013
Serializing JavaScript Promise Execution
Occasionally I have need to run a set of unrelated promises in series, for instance an object dealing with a WinRT camera API that can only execute one async operation at a time, or an object handling postMessage message events and must resolve associated async operations in the same order it received the requests. The solution is very simply to keep track of the last promise and when adding a new promise in serial add a continuation of the last promise to execute the new promise and point the last promise at the result. I encapsulate the simple solution in a simple constructor:
The only thing to watch out for is to ensure you don't pass the result of a previous promise onto a subsequent promise that is unrelated.
function PromiseExecutionSerializer() {
var lastPromise = WinJS.Promise.wrap(); // Start with an empty fulfilled promise.
this.addPromiseForSerializedExecution = function(promiseFunction) {
lastPromise = lastPromise.then(function () {
// Don't call directly so next promise doesn't get previous result parameter.
return promiseFunction();
});
}
}
The only thing to watch out for is to ensure you don't pass the result of a previous promise onto a subsequent promise that is unrelated.
Wednesday, August 7, 2013
Considerate MessagePort Usage
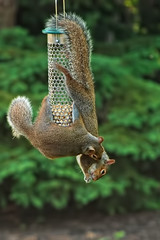
- Require a caller provided unique MessagePort
- This solves the problem but puts a lot of work on the caller who may not notice nor follow this requirement.
- Uniquely mark my messages
- To ensure I'm acting upon my own messages and not messages that happen to have similar properties as my own, I place a 'type' property on my postMessage data with a value of a URN unique to me and my JS library. Usually because its easy I use a UUID URN. There's no way someone will coincidentally produce this same URN. With this I can be sure I'm not processing someone else's messages. Of course there's no way to modify my postMessage data to prevent another library from accidentally processing my messages as their own. I can only hope they take similar steps as this and see that my messages are not their own.
- Use caller provided MessagePort only to upgrade to new unique MessagePort
- I can also make my own unique MessagePort for which only my library will have the end points. This does still require the caller to provide an initial message channel over which I can communicate my new unique MessagePort which means I still have the problems above. However it clearly reduces the surface area of the problem since I only need once message to communicate the new MessagePort.
Photo is Sharing by leezie5. Two squirrels sharing food hanging from a bird feeder. Used under Creative Commons license Attribution-NonCommercial-NoDerivs 2.0 Generic.
Labels:
DOM,
html,
javascript,
messagechannel,
postMessage,
programming,
technical
Thursday, July 25, 2013
URI functions in Windows Store Applications
Summary
The Modern SDK contains some URI related functionality as do libraries available in particular projection languages. Unfortunately, collectively these APIs do not cover all scenarios in all languages. Specifically, JavaScript and C++ have no URI building APIs, and C++ additionally has no percent-encoding/decoding APIs.
WinRT (JS and C++)
|
JS Only
|
C++ Only
|
.NET Only
| |
Parse
| ||||
Build
| ||||
Normalize
| ||||
Equality
| ||||
Relative resolution
| ||||
Encode data for including in URI property
| ||||
Decode data extracted from URI property
| ||||
Build Query
| ||||
Parse Query
|
The Windows.Foudnation.Uri type is not projected into .NET modern applications. Instead those applications use System.Uri and the platform ensures that it is correctly converted back and forth between Windows.Foundation.Uri as appropriate. Accordingly the column marked WinRT above is applicable to JS and C++ modern applications but not .NET modern applications. The only entries above applicable to .NET are the .NET Only column and the WwwFormUrlDecoder in the bottom left which is available to .NET.
Scenarios
Parse
This functionality is provided by the WinRT API Windows.Foundation.Uri in C++ and JS, and by System.Uri in .NET.
Parsing a URI pulls it apart into its basic components without decoding or otherwise modifying the contents.
var uri = new Windows.Foundation.Uri("http://example.com/path%20segment1/path%20segment2?key1=value1&key2=value2");
console.log(uri.path);// /path%20segment1/path%20segment2
WsDecodeUrl (C++)
WsDecodeUrl is not suitable for general purpose URI parsing. Use Windows.Foundation.Uri instead.
Build (C#)
URI building is only available in C# via System.UriBuilder.
URI building is the inverse of URI parsing: URI building allows the developer to specify the value of basic components of a URI and the API assembles them into a URI.
To work around the lack of a URI building API developers will likely concatenate strings to form their URIs. This can lead to injection bugs if they don’t validate or encode their input properly, but if based on trusted or known input is unlikely to have issues.
Uri originalUri = new Uri("http://example.com/path1/?query");
UriBuilder uriBuilder = new UriBuilder(originalUri);
uriBuilder.Path = "/path2/";
WsEncodeUrl (C++)
WsEncodeUrl, in addition to building a URI from components also does some encoding. It encodes non-US-ASCII characters as UTF8, the percent, and a subset of gen-delims based on the URI property: all :/?#[]@ are percent-encoded except :/@ in the path and :/?@ in query and fragment.
Accordingly, WsEncodeUrl is not suitable for general purpose URI building. It is acceptable to use in the following cases:
- You’re building a URI out of non-encoded URI properties and don’t care about the difference between encoded and decoded characters. For instance you’re the only one consuming the URI and you uniformly decode URI properties when consuming – for instance using WsDecodeUrl to consume the URI.
- You’re building a URI with URI properties that don’t contain any of the characters that WsEncodeUrl encodes.
Normalize
This functionality is provided by the WinRT API Windows.Foundation.Uri in C++ and JS and by System.Uri in .NET. Normalization is applied during construction of the Uri object.
URI normalization is the application of URI normalization rules (including DNS normalization, IDN normalization, percent-encoding normalization, etc.) to the input URI.
var normalizedUri = new Windows.Foundation.Uri("HTTP://EXAMPLE.COM/p%61th foo/");
console.log(normalizedUri.absoluteUri); // http://example.com/path%20foo/
This is modulo Win8 812823 in which the Windows.Foundation.Uri.AbsoluteUri property returns a normalized IRI not a normalized URI. This bug does not affect System.Uri.AbsoluteUri which returns a normalized URI.
Equality
This functionality is provided by the WinRT API Windows.Foundation.Uri in C++ and JS and by System.Uri in .NET.
URI equality determines if two URIs are equal or not necessarily equal.
var uri1 = new Windows.Foundation.Uri("HTTP://EXAMPLE.COM/p%61th foo/"),
uri2 = new Windows.Foundation.Uri("http://example.com/path%20foo/");
console.log(uri1.equals(uri2)); // true
Relative resolution
This functionality is provided by the WinRT API Windows.Foundation.Uri in C++ and JS and by System.Uri in .NET
Relative resolution is a function that given an absolute URI A and a relative URI B, produces a new absolute URI C. C is the combination of A and B in which the basic components specified in B override or combine with those in A under rules specified in RFC 3986.
var baseUri = new Windows.Foundation.Uri("http://example.com/index.html"),
relativeUri = "/path?query#fragment",
absoluteUri = baseUri.combineUri(relativeUri);
console.log(baseUri.absoluteUri); // http://example.com/index.html
console.log(absoluteUri.absoluteUri); // http://example.com/path?query#fragment
Encode data for including in URI property
This functionality is available in JavaScript via encodeURIComponent and in C# via System.Uri.EscapeDataString. Although the two methods mentioned above will suffice for this purpose, they do not perform exactly the same operation.
Additionally we now have Windows.Foundation.Uri.EscapeComponent in WinRT, which is available in JavaScript and C++ (not C# since it doesn’t have access to Windows.Foundation.Uri). This is also slightly different from the previously mentioned mechanisms but works best for this purpose.
Encoding data for inclusion in a URI property is necessary when constructing a URI from data. In all the above cases the developer is dealing with a URI or substrings of a URI and so the strings are all encoded as appropriate. For instance, in the parsing example the path contains “path%20segment1” and not “path segment1”. To construct a URI one must first construct the basic components of the URI which involves encoding the data. For example, if one wanted to include “path segment / example” in the path of a URI, one must percent-encode the ‘ ‘ since it is not allowed in a URI, as well as the ‘/’ since although it is allowed, it is a delimiter and won’t be interpreted as data unless encoded.
If a developer does not have this API provided they can write it themselves. Percent-encoding methods appear simple to write, but the difficult part is getting the set of characters to encode correct, as well as handling non-US-ASCII characters.
var uri = new Windows.Foundation.Uri("http://example.com" +
"/" + Windows.Foundation.Uri.escapeComponent("path segment / example") +
"?key=" + Windows.Foundation.Uri.escapeComponent("=&?#"));
WsEncodeUrl (C++)
In addition to building a URI from components, WsEncodeUrl also percent-encodes some characters. However the API is not recommend for this scenario given the particular set of characters that are encoded and the convoluted nature in which a developer would have to use this API in order to use it for this purpose.
There are no general purpose scenarios for which the characters WsEncodeUrl encodes make sense: encode the %, encode a subset of gen-delims but not also encode the sub-delims. For instance this could not replace encodeURIComponent in a C++ version of the following code snippet since if ‘value’ contained ‘&’ or ‘=’ (both sub-delims) they wouldn’t be encoded and would be confused for delimiters in the name value pairs in the query:
"http://example.com/?key=" + Windows.Foundation.Uri.escapeComponent(value)
Since WsEncodeUrl produces a string URI, to obtain the property they want to encode they’d need to parse the resulting URI. WsDecodeUrl won’t work because it decodes the property but Windows.Foundation.Uri doesn’t decode. Accordingly the developer could run their string through WsEncodeUrl then Windows.Foundation.Uri to extract the property.
Decode data extracted from URI property
This functionality is available in JavaScript via decodeURIComponent and in C# via System.Uri.UnescapeDataString. Although the two methods mentioned above will suffice for this purpose, they do not perform exactly the same operation.
Additionally we now also have Windows.Foundation.Uri.UnescapeComponent in WinRT, which is available in JavaScript and C++ (not C# since it doesn’t have access to Windows.Foundation.Uri). This is also slightly different from the previously mentioned mechanisms but works best for this purpose.
Decoding is necessary when extracting data from a parsed URI property. For example, if a URI query contains a series of name and value pairs delimited by ‘=’ between names and values, and by ‘&’ between pairs, one must first parse the query into name and value entries and then decode the values. It is necessary to make this an extra step separate from parsing the URI property so that sub-delimiters (in this case ‘&’ and ‘=’) that are encoded will be interpreted as data, and those that are decoded will be interpreted as delimiters.
If a developer does not have this API provided they can write it themselves. Percent-decoding methods appear simple to write, but have some tricky parts including correctly handling non-US-ASCII, and remembering not to decode .
In the following example, note that if unescapeComponent were called first, the encoded ‘&’ and ‘=’ would be decoded and interfere with the parsing of the name value pairs in the query.
var uri = new Windows.Foundation.Uri("http://example.com/?foo=bar&array=%5B%27%E3%84%93%27%2C%27%26%27%2C%27%3D%27%2C%27%23%27%5D");
uri.query.substr(1).split("&").forEach(
function (keyValueString) {
var keyValue = keyValueString.split("=");
console.log(Windows.Foundation.Uri.unescapeComponent(keyValue[0]) + ": " + Windows.Foundation.Uri.unescapeComponent(keyValue[1]));
// foo: bar
// array: ['ㄓ','&','=','#']
});
WsDecodeUrl (C++)
Since WsDecodeUrl decodes all percent-encoded octets it could be used for general purpose percent-decoding but it takes a URI so would require the dev to construct a stub URI around the string they want to decode. For example they could prefix “http:///#” to their string, run it through WsDecodeUrl and then extract the fragment property. It is convoluted but will work correctly.
Parse Query
The query of a URI is often encoded as application/x-www-form-urlencoded which is percent-encoded name value pairs delimited by ‘&’ between pairs and ‘=’ between corresponding names and values.
In WinRT we have a class to parse this form of encoding using Windows.Foundation.WwwFormUrlDecoder. The queryParsed property on the Windows.Foundation.Uri class is of this type and created with the query of its Uri:
var uri = Windows.Foundation.Uri("http://example.com/?foo=bar&array=%5B%27%E3%84%93%27%2C%27%26%27%2C%27%3D%27%2C%27%23%27%5D");
uri.queryParsed.forEach(
function (pair) {
console.log("name: " + pair.name + ", value: " + pair.value);
// name: foo, value: bar
// name: array, value: ['ㄓ','&','=','#']
});
console.log(uri.queryParsed.getFirstValueByName("array")); // ['ㄓ','&','=','#']
The QueryParsed property is only on Windows.Foundation.Uri and not System.Uri and accordingly is not available in .NET. However the Windows.Foundation.WwwFormUrlDecoder class is available in C# and can be used manually:
Uri uri = new Uri("http://example.com/?foo=bar&array=%5B%27%E3%84%93%27%2C%27%26%27%2C%27%3D%27%2C%27%23%27%5D");
WwwFormUrlDecoder decoder = new WwwFormUrlDecoder(uri.Query);
foreach (IWwwFormUrlDecoderEntry entry in decoder)
{
System.Diagnostics.Debug.WriteLine("name: " + entry.Name + ", value: " + entry.Value);
// name: foo, value: bar
// name: array, value: ['ㄓ','&','=','#']
}
Build Query
To build a query of name value pairs encoded as application/x-www-form-urlencoded there is no WinRT API to do this directly. Instead a developer must do this manually making use of the code described in “Encode data for including in URI property”.
In terms of public releases, this property is only in the RC and later builds.
For example in JavaScript a developer may write:
var uri = new Windows.Foundation.Uri("http://example.com/"),
query = "?" + Windows.Foundation.Uri.escapeComponent("array") + "=" + Windows.Foundation.Uri.escapeComponent("['ㄓ','&','=','#']");
console.log(uri.combine(new Windows.Foundation.Uri(query)).absoluteUri); // http://example.com/?array=%5B'%E3%84%93'%2C'%26'%2C'%3D'%2C'%23'%5D
Labels:
c#,
c++,
javascript,
technical,
uri,
windows,
windows-runtime,
windows-store
Thursday, July 18, 2013
C++ constructor member initializers run in member declaration order
TL;DR: Keep your C++ class member declaration order the same as your constructor member initializers order.
C++ guarantees that the member initializers in a constructor are called in order. However the order in which they are called is the order in which the associated members are declared in the class, not the order in which they appear in the member initializer list. For instance, take the following code. I would have thought it would print "three, one, two", but in fact it prints, "one, two, three".
#include "stdafx.h"
#include <iostream>
class PrintSomething {
public:
PrintSomething(const wchar_t *name) { std::wcout << name << std::endl; }
};
class NoteOrder {
public:
// This order doesn't matter.
NoteOrder() : three(L"three"), one(L"one"), two(L"two") { }
PrintSomething one;
PrintSomething two;
PrintSomething three;
};
int wmain(const int argc, const wchar_t* argv[])
{
NoteOrder note; // Prints one, two, three, not three, one, two!
return 0;
}
Subscribe to:
Posts (Atom)